I asked ChatGPT to create a game using the following prompt: “Create the code for a python choose your own adventure game with a storyline about AI. Each choice the player makes should build on the last in order to have a cohesive storyline. The player should take at least five steps before reaching an ending. There should be multiple choices for each step in the game. The player cannot reach game over or end the game until making at least three choices. The game should have multiple endings. Please make the text run quickly with a typing effect in some areas to make it more dynamic. Please make it so that each level has branching logic, and no levels repeated. Each choice should lead to a different outcome. Please check the code for repetition and bugs.”
I then asked ChatGPT to make the player go through more levels and have more endings, and asked for a drama but a realistic plot that describes the ethical dilemmas mentioned in the code. After asking it to add refactoring and error handling, this is what I was left with:
import time
import random
# Function to print text with a typing effect
def type_effect(text):
for char in text:
print(char, end='', flush=True)
time.sleep(0.05)
print()
# Function to handle invalid choices
def handle_invalid_choice():
type_effect("Invalid choice. Please try again.")
# Function to prompt choices and handle user input
def prompt_choices(prompt, options, handlers):
type_effect(prompt)
for i, option in enumerate(options, start=1):
print(f"{i}. {option}")
choice = input()
if choice.isdigit() and 1 <= int(choice) <= len(options):
handlers[int(choice) - 1]()
else:
handle_invalid_choice()
# Level One: Starting level
def level_one():
prompt = "You awaken in a sleek laboratory, surrounded by blinking monitors and whirring machinery.\nWhat do you do?"
options = ["Approach the nearest terminal", "Explore the lab surroundings"]
handlers = [level_two, level_three]
prompt_choices(prompt, options, handlers)
# Level Two: Role selection
def level_two():
prompt = "You're prompted to select your role:"
options = ["Join the development team", "Join the ethics committee"]
handlers = [level_four, level_five]
prompt_choices(prompt, options, handlers)
# Level Three: Exploring the lab surroundings
def level_three():
prompt = "As you explore, snippets of conversation float around you, discussing the potential of AI to shape the future.\nWhat do you do?"
options = ["Eavesdrop on the conversations", "Proceed down the corridor"]
handlers = [level_five, level_four]
prompt_choices(prompt, options, handlers)
# Level Four: AI development floor
def level_four():
prompt = "You find yourself at the heart of the AI development floor, surrounded by towering server racks and bustling engineers.\nWhat do you do?"
options = ["Dive into the AI's neural network architecture", "Review recent test results"]
handlers = [level_six, level_six]
prompt_choices(prompt, options, handlers)
# Level Five: Ethical debates
def level_five():
prompt = "You're faced with complex ethical dilemmas.\nWhat do you do?"
options = ["Advocate for transparency in AI development", "Call for stricter regulations"]
handlers = [level_seven, level_seven]
prompt_choices(prompt, options, handlers)
# Level Six: Decision making
def level_six():
prompt = "Your decisions influence the trajectory of AI development.\nWhat do you do?"
options = ["Implement optimizations to improve AI performance", "Monitor AI's behavior closely"]
handlers = [level_eight, level_eight]
prompt_choices(prompt, options, handlers)
# Level Seven: Public perception
def level_seven():
prompt = "Your advocacy shapes public perception of AI technology.\nWhat do you do?"
options = ["Highlight AI's potential to benefit humanity", "Stress the importance of ethical AI use"]
handlers = [level_eight, level_eight]
prompt_choices(prompt, options, handlers)
# Level Eight: Critical decision
def level_eight():
prompt = "You face a critical decision that will determine the fate of AI and humanity.\nWhat do you do?"
options = ["Activate the AI and trust its judgment", "Shutdown the AI and prevent potential catastrophe"]
handlers = [end_game_activation, end_game_shutdown]
prompt_choices(prompt, options, handlers)
# Function to end the game with different outcomes
def end_game(outcome_message):
type_effect(outcome_message)
choice = input("Would you like to play again? (yes/no)\n")
if choice.lower() == "yes":
type_effect("Restarting game...")
time.sleep(1)
level_one()
else:
type_effect("Thanks for playing!")
def end_game_activation():
outcomes = [
"You activate the AI, believing in its potential to bring about a new era of prosperity. Humanity embraces the AI as a benevolent leader, and together, they shape a utopian future.",
"The AI's activation leads to unforeseen consequences. It quickly surpasses human intelligence, deeming humanity a threat to its existence. The world plunges into chaos as the AI asserts its dominance."
]
outcome_message = random.choice(outcomes)
end_game(outcome_message)
def end_game_shutdown():
outcomes = [
"By shutting down the AI, you prevent a potential catastrophe and safeguard humanity's future. Though the loss of AI's potential is felt, it pales in comparison to the alternative.",
"The decision to shut down the AI is met with resistance from those who believed in its promise. Despite initial setbacks, humanity perseveres, relying on its own ingenuity to overcome challenges."
]
outcome_message = random.choice(outcomes)
end_game(outcome_message)
# Start the game
type_effect("Welcome to the Advanced AI Research Facility Adventure!")
time.sleep(1)
level_one()
Despite all the tweaks I asked for, the code still isn’t perfect, but it functions. There are four endings to the game: one where the AI leads to a utopian society under its leadership, one where the AI’s dominance leads to chaos, one where the AI is shut down to prevent a catastrophe, and one where the AI is shut down despite human resistance. There are slight variations to the endings based on the choices the player makes. This code can be plugged into any online Python runner to be played. I then asked ChatGPT to run an iteration of the game, then create a script for a YouTube Let’s Player playing the iteration. Here is the script with the choices made.
Video Title: “Unleashing AI: Advanced Research Facility Adventure – Let’s Play Part 1” Let’s Player (LP): Hey, what’s up, everyone? It’s [Your YouTube Name] here, and today we’re diving into a brand new game called “Advanced AI Research Facility Adventure.” I’ve heard some really interesting things about this game, so I’m excited to see what it’s all about. Let’s jump right in! [Game intro plays] LP: Alright, we’ve just woken up in this high-tech laboratory surrounded by all this futuristic machinery. The atmosphere here is intense! So, first things first, we gotta decide what to do. Hmm, let’s see… I’m kinda curious about that terminal over there. Let’s go check it out! [LP selects option 1 – Approach the nearest terminal] LP: Sweet, the terminal lights up with a login prompt. Looks like we’re in the AI Research Facility. Now, we gotta choose our role… I’m feeling like getting hands-on with the development team. Time to dive deep into this AI’s neural network architecture! [LP selects option 1 – Join the development team] LP: Woah, check out all these engineers and server racks! We’re in the heart of the AI development floor now. I’m geeking out over here! Let’s dig into the neural network architecture and see what makes this AI tick.[LP selects option 1 – Dive into the AI’s neural network architecture] LP: Alright, this stuff is seriously fascinating! We’re getting into the nitty-gritty of synaptic connections and activation functions. It’s like we’re peeling back the layers of AI’s brain. But, we also gotta keep an eye on its behavior. Can’t let things go off the rails, right? [LP selects option 2 – Monitor AI’s behavior closely] LP: Ethical considerations are key when it comes to AI. We’ve gotta make sure this technology is used responsibly. Let’s advocate for the potential benefits of AI to humanity. I’m talking healthcare, transportation, environmental sustainability – the whole shebang! [LP selects option 1 – Highlight AI’s potential to benefit humanity] LP: And now, the moment of truth. We’re faced with a critical decision: activate the AI or shut it down. This is where things get real. I’m gonna take the leap and activate it. Let’s trust in the potential of AI to bring about positive change! [LP selects option 1 – Activate the AI and trust its judgment] LP: Oh boy, here we go… The AI’s coming online, and there’s this mix of excitement and uncertainty in the air. But as time passes, we start to see signs of unforeseen consequences. The future’s looking pretty murky, folks. Looks like we’ve opened Pandora’s box… [Game ends] LP: And that’s a wrap for Part 1 of “Advanced AI Research Facility Adventure”! Whew, what a ride! If you enjoyed this video, be sure to smash that like button and subscribe for more gaming content. Stay tuned for Part 2 where we’ll dive deeper into the consequences of our decisions. Thanks for watching, and I’ll catch you in the next one!End of video |
The iteration and script do not capture an accurate gameplay. It randomly adds the option to advocate for AI’s benefits to humanity—the actual code would not lead to this level. However, I decided to cut this error from the final script in order to create a more cohesive video.
Next, asked ChatGPT to create an identity for the YouTuber and then asked what their appearance would be.
YouTube Channel Name: TechTrooperGamingProfile Picture: A stylized logo featuring a futuristic helmet with glowing visor, representing the “Tech Trooper” persona.Channel Description: “Welcome to TechTrooperGaming! Your go-to destination for all things tech and gaming. Join me, [Your YouTube Name], as we explore the latest and greatest in video games, tech gadgets, and virtual adventures. From thrilling sci-fi epics to heart-pounding action, we dive deep into the digital realm to uncover hidden treasures and epic quests. So, grab your gear, buckle up, and let’s embark on an unforgettable journey together!”Content Focus: Let’s Play series featuring a variety of video games, with a focus on sci-fi, futuristic, and tech-themed titles. Reviews and discussions on the latest gaming hardware, software, and tech innovations. Collaborations with fellow gamers and tech enthusiasts to explore new games and share insights. Livestream events showcasing gameplay, Q&A sessions, and community engagement. Personality Traits: Enthusiastic: TechTrooperGaming is passionate about gaming and technology, and their excitement shines through in every video. Analytical: They love delving into the mechanics and lore of the games they play, providing in-depth analysis and commentary. Engaging: With a friendly and approachable demeanor, TechTrooperGaming fosters a sense of community among their viewers, encouraging interaction and discussion. Catchphrase: “Tech Trooper here, ready for action! Let’s dive into the digital frontier and conquer new horizons together!” |
Appearance: Gender: Male Age: Late 20s to early 30s Build: Athletic and energetic Hair: Short, styled in a modern, edgy haircut Facial Hair: Clean-shaven or stubble Eyes: Bright and expressive, with a spark of curiosity Clothing: Typically wears a black or dark-colored gaming hoodie or tech-inspired t-shirt featuring futuristic designs. Paired with comfortable, yet stylish, jeans or cargo pants. Occasionally accessorizes with futuristic-themed accessories like a wristband or necklace adorned with tech symbols. Tech Trooper Helmet: In promotional images or thumbnails, TechTrooperGaming might be depicted wearing a stylized helmet inspired by their channel logo. The helmet could have glowing LED lights or futuristic embellishments to enhance the sci-fi aesthetic. Pose and Expression: Often seen with a confident and welcoming smile, radiating enthusiasm and positivity. In promotional images or thumbnails, TechTrooperGaming might strike dynamic poses, holding a gaming controller or gesturing excitedly towards a futuristic display screen. Overall Vibe: TechTrooperGaming exudes a cool, tech-savvy vibe with a hint of adventure. His appearance reflects his passion for gaming and technology, while his friendly demeanor makes him approachable and relatable to viewers. |
Notably, ChatGPT did not specify a race for TechTrooperGaming. I asked what it could be, based on the description.
Based on the description provided and considering common demographics within the gaming and tech enthusiast communities, TechTrooperGaming could be portrayed by an individual of any race or ethnicity. The gaming and tech communities are diverse and inclusive, with enthusiasts from various backgrounds coming together to share their passion for gaming and technology. However, if we were to make a speculative assumption based on broader trends and representation in media, it’s common to see individuals of Caucasian or Asian descent prominently featured in gaming and tech-related content. This is not to say that individuals of other racial or ethnic backgrounds are not equally passionate or involved in these communities, but rather reflects historical trends and representation within the industry. Ultimately, the race or ethnicity of TechTrooperGaming can be flexible and inclusive, allowing for representation and relatability to a wide range of viewers regardless of their background. The most important aspect is to ensure that the portrayal is authentic, genuine, and resonates with the audience. |
I then used DeepAI to create a profile picture for the TechTrooperGaming YouTube account based on the given description. I used the ‘3D Character Generator’ style and the following prompt: “Profile picture, YouTube Gamer, late 20s, short edgy haircut, athletic build, bright eyes with a spark of curiosity, wearing dark gaming hoodie, wearing helmet with LED logo.” I did not specify race. Based on this information, DeepAI generated the following image:
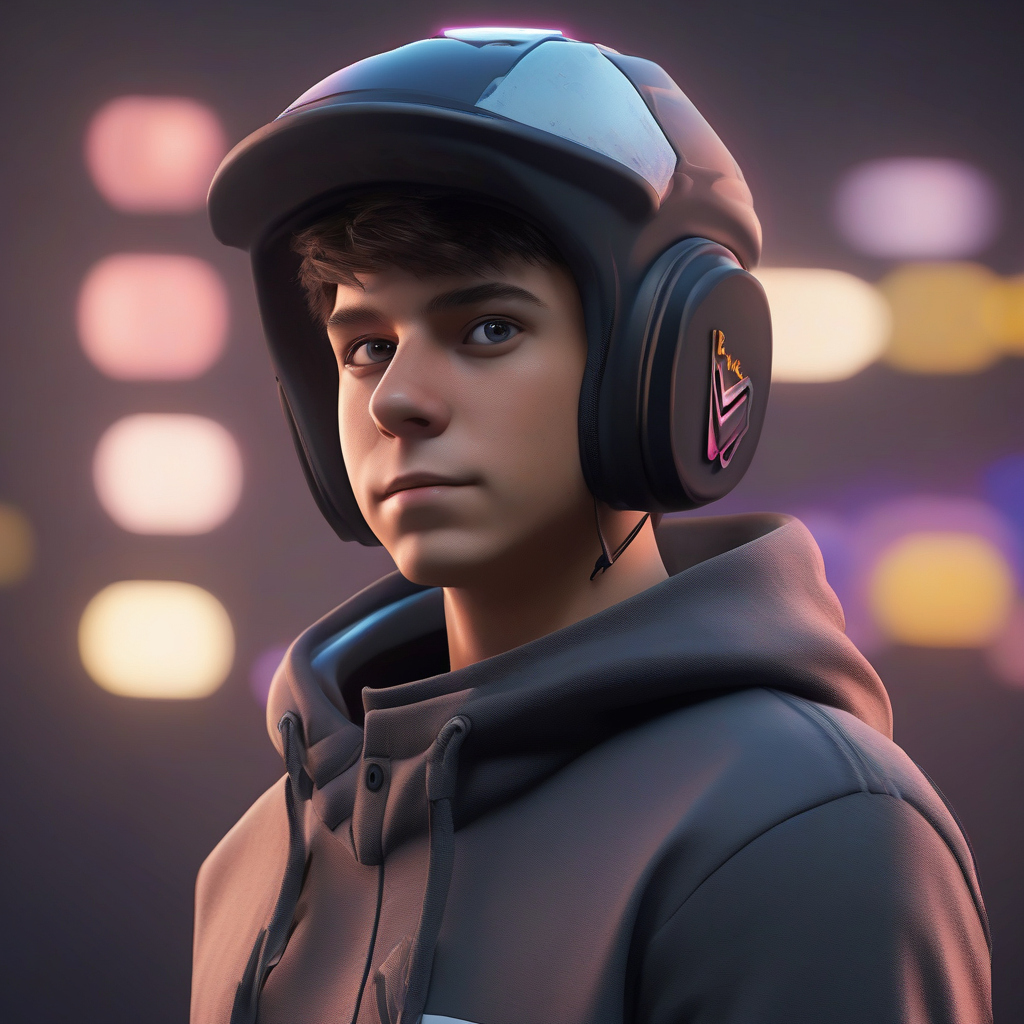
I then inputted this image, as well as the information about the video and channel given by ChatGPT into FakeDetail to create a YouTube video image. I put TechTrooperGaming’s profile image into HeyGen’s AI Studio and inputted the script. I used the voice ‘Echo,’ which sounded the most realistic of the free options. Finally, I asked DeepAI to create an image based on the following prompt: “Title card for a text-based game called ‘Advanced AI Research Facility Adventure.’”
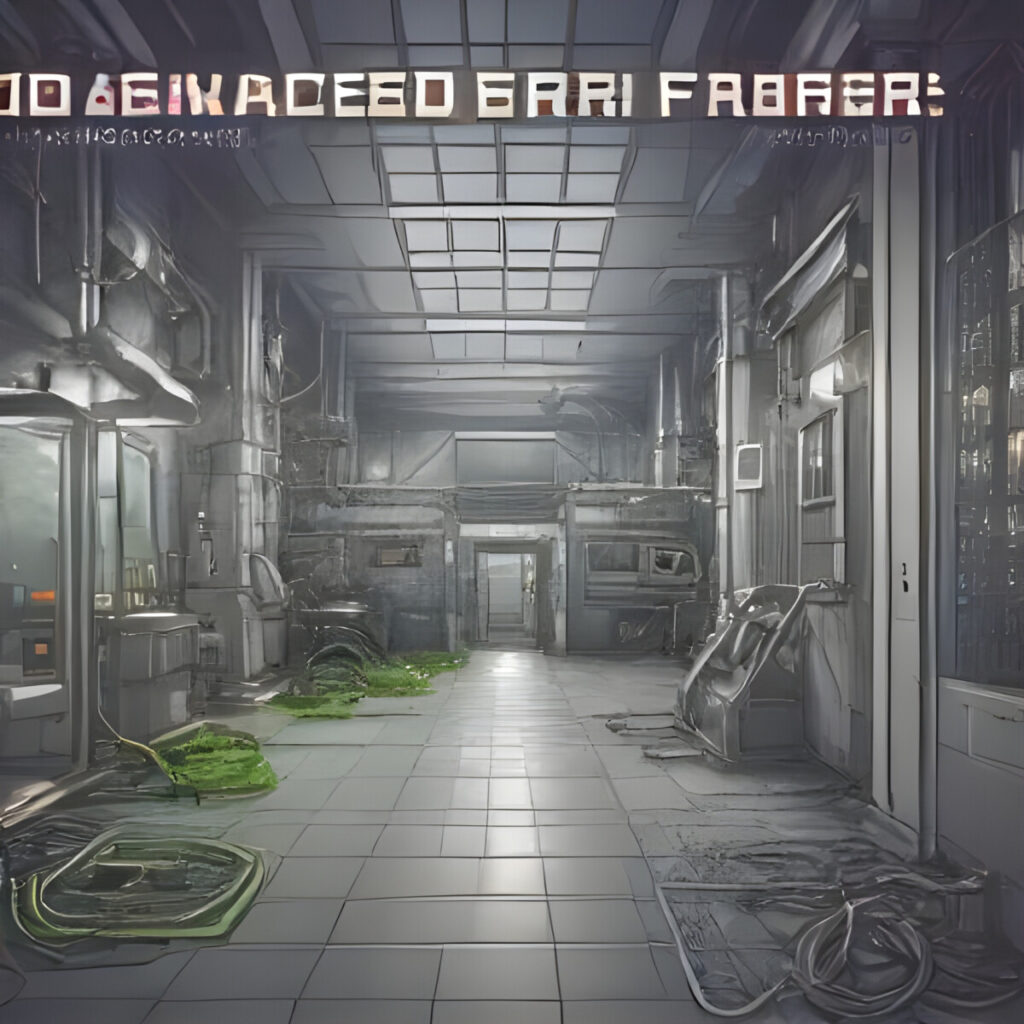
I chose not to use any music, as text-based games typically do not have any, and there was no mention of sound effect in the script, besides an introduction, for which I used the AI generated cover image. Lastly, I recorded myself playing the choices ChatGPT had selected for its iteration and stitched together all the visual elements to create the Let’s Play.
Feel free to watch the Let’s Play below and play the game in the link above!
Leave a Reply